Python Crypto Rsa Key Generate
The RSA private key format includes all the public elements. When you get the private key you really have both the private and public key. This is described in PKCS#1 (the leading RSA standard); private key format is an encoded ASN.1 structure which contains. Openssl genrsa -des3 -out private.pem 2048 That generates a 2048-bit RSA key pair, encrypts them with a password you provide and writes them to a file. You need to next extract the public key file. You will use this, for instance, on your web server to encrypt content so that it can only be read with the private key. OpenSSL can generate several kinds of public/private keypairs. RSA is the most common kind of keypair generation. Other popular ways of generating RSA public key / private key pairs include PuTTYgen and ssh-keygen. Openssl rsa key pair generation.
Encrypt data with AES¶
Crypto.PublicKey package¶. In a public key cryptography system, senders and receivers do not use the same key. Instead, the system defines a key pair, with one of the keys being confidential (private) and the other not (public). Edit: I just realized that pycrypto already has this: import os from Crypto.PublicKey import RSA key = RSA.generate(2048, os.urandom) print key.exportKey('OpenSSH'). The following are code examples for showing how to use Crypto.PublicKey.RSA.They are from open source Python projects. You can vote up the examples you like or vote down the ones you don't like. Oct 02, 2015 SSH Config and crypto key generate RSA command. Use this command to generate RSA key pairs for your Cisco device (such as a router). Keys are generated in pairs–one public RSA key and one private RSA key. If your router already has RSA keys when you issue this command, you will be warned and prompted to replace the existing keys with new keys. Crypto — Generic cryptographic module. Check the consistency of an RSA private key. This is the Python equivalent of OpenSSL’s RSAcheckkey. Returns: True if key is consistent. Generate a key pair of the given type, with the given number of bits. RSA encryption and decryption in Python (3) I need help using RSA encryption and decryption in Python. I am creating a private/public key pair, encrypting a message with keys and writing message to a file. Then I am reading ciphertext from file and decrypting text using key. Oct 24, 2019 An example of asymmetric encryption in python using a public/private keypair - utilizes RSA from PyCrypto library - RSAexample.py. Privatekey = RSA. Generate (moduluslength, Random. Read) publickey = privatekey. Look in your python directory for /Lib/site-packages/crypto and change it to Crypto. (Capital C) This.
The following code generates a new AES128 key and encrypts a piece of data into a file.We use the EAX mode because it allows the receiver to detect anyunauthorized modification (similarly, we could have used other authenticatedencryption modes like GCM, CCM or SIV).
You use your server to generate the associated private key file where the CSR was created.You need both the public key and private keys for an SSL certificate to work properly on any system. SSLSupportDesk is part of Acmetek who is a trusted advisor of security solutions and services. Generating private key and ssl signed certificate. If you are looking for security look no further. They provide comprehensive security solutions that include Encryption & Authentication (SSL), Endpoint Protection, Multi-factor Authentication, PKI/Digital Signing Certificates, DDOS, WAF and Malware Removal.
At the other end, the receiver can securely load the piece of data back (if they know the key!).Note that the code generates a ValueError
exception when tampering is detected.
Crypto Key Zeroize Rsa
Generate an RSA key¶

Generate Rsa Key Command
The following code generates a new RSA key pair (secret) and saves it into a file, protected by a password.We use the scrypt key derivation function to thwart dictionary attacks.At the end, the code prints our the RSA public key in ASCII/PEM format:
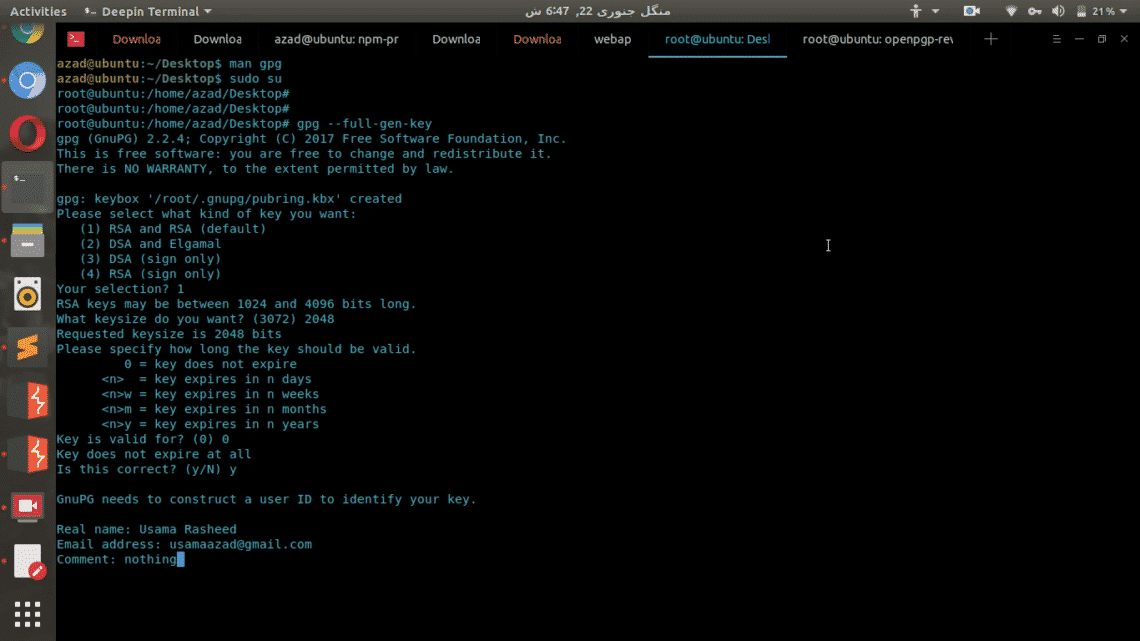
The following code reads the private RSA key back in, and then prints again the public key:
Generate public key and private key¶
The following code generates public key stored in receiver.pem
and private key stored in private.pem
. These files will be used in the examples below. Every time, it generates different public key and private key pair.
Encrypt data with RSA¶
The following code encrypts a piece of data for a receiver we have the RSA public key of.The RSA public key is stored in a file called receiver.pem
.
Since we want to be able to encrypt an arbitrary amount of data, we use a hybrid encryption scheme.We use RSA with PKCS#1 OAEP for asymmetric encryption of an AES session key.The session key can then be used to encrypt all the actual data.
As in the first example, we use the EAX mode to allow detection of unauthorized modifications.
The receiver has the private RSA key. They will use it to decrypt the session keyfirst, and with that the rest of the file: